Understanding Task and async-await in C#: A Fun and Simple Guide
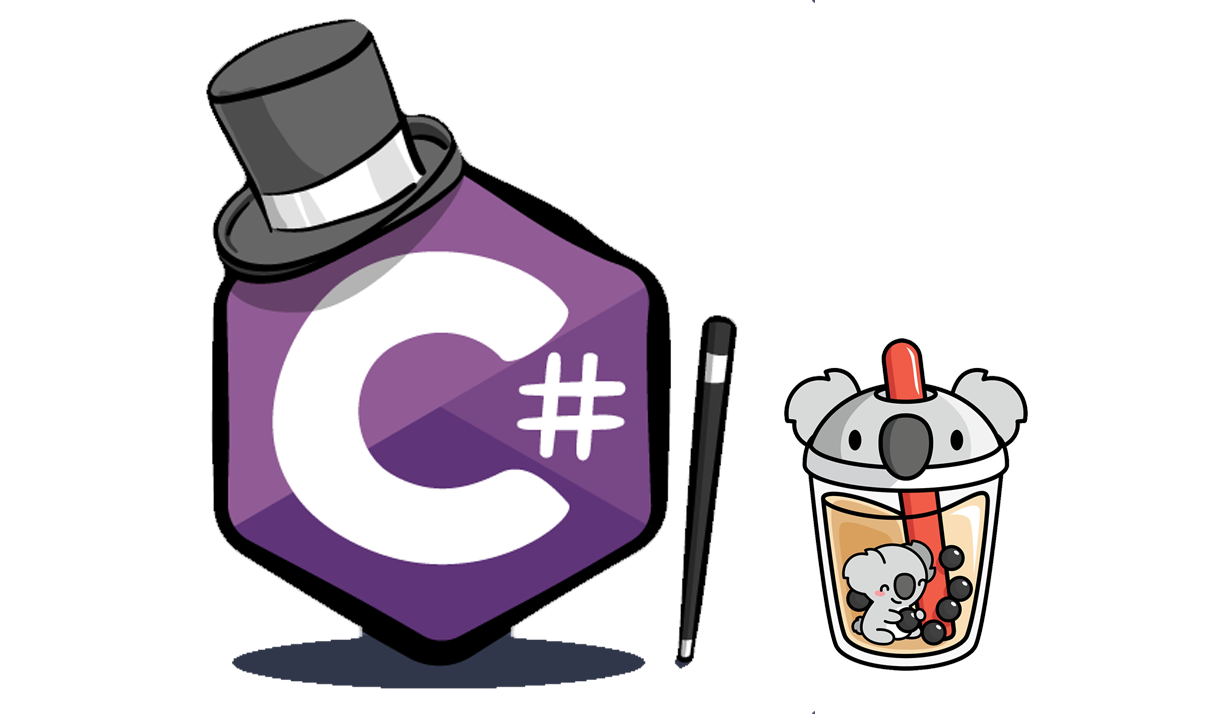
Have you ever wondered how asynchronous programming works in C#? Let’s break it down with relatable and fun examples—like buying bubble tea (because who doesn’t love bubble tea? 🍹). Let’s dive in!
I. What Are Task
and async-await
?
Imagine you're buying bubble tea 🍹:
Synchronous (Traditional) Way:
- You queue up.
- You wait for your tea to be made 🍶.
- Finally, you take the tea and leave 🍹.
→ You can’t do anything else while waiting.
Asynchronous with Task
:
Task<Tea> tea = BuyTea();
// Like taking a queue number, so you’re free to do other things.
Asynchronous with async-await
:
var tea = await BuyTea();
// It’s like asking your best friend to buy it for you.
// `await` = "Wait for your friend to return with the tea before continuing."
II. Understanding Task.WhenAll
: The Power of Teamwork
Let's have a party!!
Ever tried delegating tasks to multiple friends? (like a boss).
Example:
// Sequential tasks
await Friend1BuyTea(); // Wait for Friend 1 to finish.
await Friend2BuySnacks(); // Then wait for Friend 2.
// Concurrent tasks
await Task.WhenAll(
Friend1BuyTea(),
Friend2BuySnacks()
); // Both friends work simultaneously. Faster and efficient!
UI Thread: Your Friendly Cashier
Imagine you're a cashier at a bubble tea shop 🍹🏪:
- UI Thread = The Cashier
You handle customer orders (updates to the UI). If you leave the counter to make tea (heavy tasks), no one serves the customers, and the queue grows. - async-await = The Helper
You delegate heavy work to the kitchen (background threads) while you continue serving customers.
When Should You Use Task
?
- When running multiple tasks simultaneously.
- When you need to cancel tasks mid-way.
- When you want to track progress or completion of tasks.
Key Examples and Good Practices
Sequential vs Parallel Tasks:
// Sequential
var coffee = await BrewCoffee();
var tea = await BrewTea();
// Parallel
var coffeeTask = BrewCoffee();
var teaTask = BrewTea();
await Task.WhenAll(coffeeTask, teaTask); // Brew both at the same time.
Avoid Blocking the UI:
// BAD: Blocks the UI
var result = BrewCoffee().Result;
// GOOD: Doesn't block the UI
var result = await BrewCoffee();
Thread Pool vs UI Thread: How Work is Divided
Think of a restaurant:
- UI Thread = Front Staff (Cashier)
Handles customer interactions like updating menus or tables. - Thread Pool = Kitchen Staff
Prepares orders in the background, allowing front staff to focus on serving customers.
Example:
public class Form1 : Form
{
public async void ButtonClick(object sender, EventArgs e)
{
label1.Text = "Preparing..."; // UI Thread updates UI
var coffee = await Task.Run(() => {
// Background work in Thread Pool
return "Coffee is ready!";
});
label1.Text = coffee; // Back on UI Thread
}
}
Bonus: ConfigureAwait and Thread Management
Tasks aren’t always tied to the UI Thread:
// Switches back to the UI Thread after await
await DoHeavyWork();
// Stays on the current thread (not UI)
await DoHeavyWork().ConfigureAwait(false);
The Takeaway
Asynchronous programming with Task
and async-await
is like delegating work smartly. It makes your app more efficient, responsive, and avoids blocking crucial operations (like keeping the UI alive).
💡 Tip: Start small—learn the basics like await
and Task.WhenAll
. Over time, dive into advanced concepts like thread pools and ConfigureAwait
. Just like learning to order your favorite bubble tea, it takes a bit of practice, but it’s worth it!
Share this with your friends if it is helpful, say ya 😎!